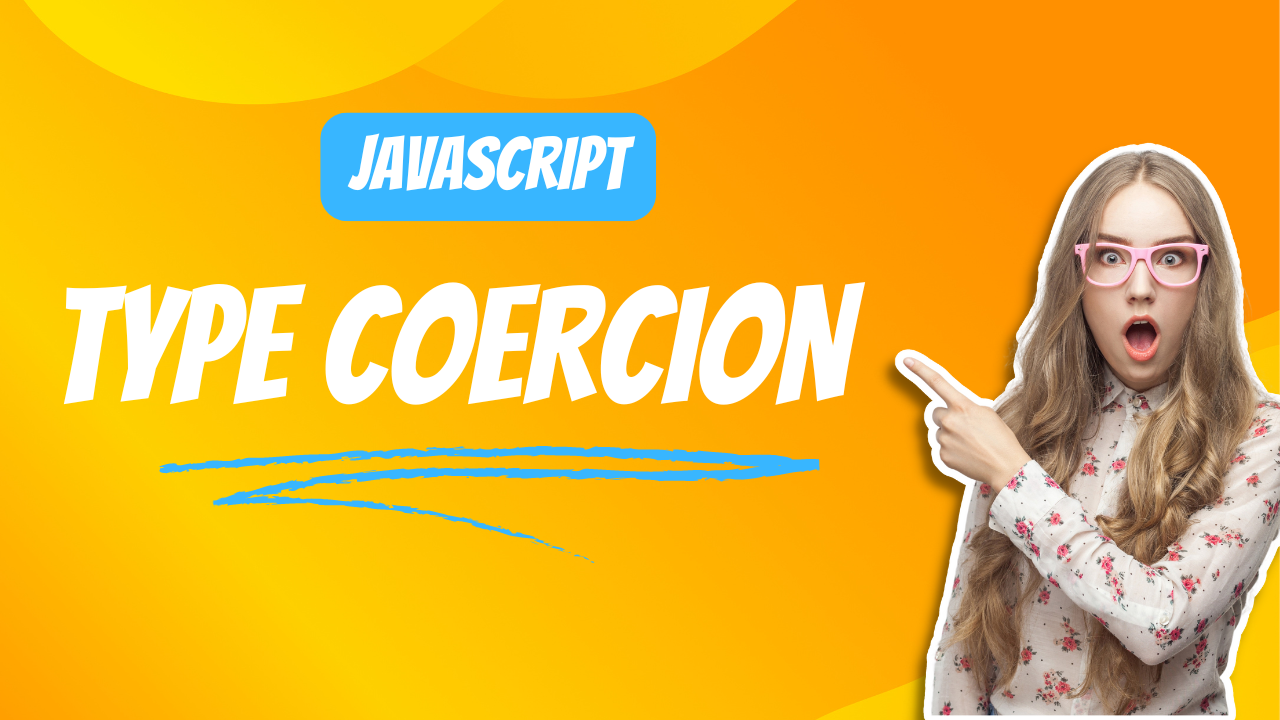
Unlike coercion, type conversion is an explicit process where a programmer deliberately changes a value from one type to another. JavaScript provides functions like Number()
, String()
, and Boolean()
to facilitate such conversions.
For example, Number('5')
converts the string '5'
into the numeric value 5
. Similarly, String(2)
converts the number 2
into the string '2'
.
What is JavaScript Coercion?
JavaScript coercion, also known as type coercion, refers to the implicit conversion of values from one data type to another during code execution. This automatic transformation is often performed to facilitate operations that involve mixed data types, such as adding a string to a number. Lets understand it by different examples.
1. String and Number Coercion
let result = '5' + 10;
console.log(result); // Output: "510"
Explanation: When a string and a number are added using the `+`
operator, JavaScript coerces the number to a string, resulting in string concatenation.
2. Boolean and Number Coercion
let result = true + 1;
console.log(result); // Output: 2
Explanation: The boolean true
is coerced into the number 1
, and then it’s added to the number 1
, resulting in 2
.
3. Number and String Comparison
let result = '5' > 3;
console.log(result); // Output: true
Explanation: The string '5'
is coerced into the number 5
, and then the comparison is made between two numbers.
4. Boolean and String Coercion
let result = 'false' == false;
console.log(result); // Output: false
Explanation: The string 'false'
is not coerced into a boolean false
. Instead, when using ==
, the string 'false'
remains a string, and false
is coerced to 0
. Since 'false'
is not 0
, the comparison returns false
.
5. Null and Undefined Comparison
let result = null == undefined;
console.log(result); // Output: true
Explanation: null
and undefined
are loosely equal (==
), so JavaScript coerces both to a similar internal type, making the comparison return true
. However, they are not strictly equal (===
).
In summary, understanding JavaScript coercion helps manage unintentional data type changes, while type conversion offers precise control over how values are transformed
Leave a Reply